Many websites give their users a custom subdomain for their profiles or pages, so instead of accessing the user’s profile at http://website.com/users/50, the user can access his profile page at http://username.website.com which is much better. In this post, we will see how to make dynamic Laravel subdomain routing easily. First you need to configure DNS. For this, You must have access to DNS server settings and apache web server settings. First, you need to add an A record with an asterisk for the subdomain like this:
* IN A 192.168.1.5
You should replace the IP address with your IP address.
Configure Web server
Open apache web server configuration file httpd.conf and add a VirtualHost like this:
Let’s assume that we have the users with the name field which will contain the user’s name.
Now we will create our route.
Route::get('/', function () {
$url = parse_url(URL::all());
$domain = explode('.', $url['host']);
$subdomain = $domain[0];
$name = DB::table('users')->where('name', $subdomain)->get();
dd($name);
// write the rest of your code.
});
First, we explode the URL and extract the host from it, then we get the subdomain part.
Then we search for a username in the users table that matches the extracted subdomain.
You can check if no user found, redirect to another page or give him an error message or whatever.
Now if you try to visit any user subdomain like http://likegeeks.website.com, you should see the user’s name without problems.
Keep in mind that the user that you are visiting his subdomain MUST be present in the database.
Any user added to the database will have his subdomain automatically without a headache.
If you don’t have access to your web server configuration like using shared hosting or so, you can’t achieve the same functionality using htaccess redirection.
Multiple Routes in Subdomain
In the above example, we use a single route to deal with the subdomain, but you can use many routes with a subdomain.
You can use routes groups to achieve this:
Route::group(array('domain' => '{subdomain}.website.com'), function () {
Route::get('/', function ($subdomain) {
$name = DB::table('users')->where('name', $subdomain)->get();
dd($name);
});
});
As you see, Laravel subdomain routing is very easy to implement.
I hope you find the post useful. Keep coming back.
Thank you.
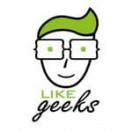